Here we’ll see how to write C program to toggle all bits or a particular bit or all but a particular bit of a number.
Toggle All Bits of a Number
We can toggle all bits of a number using NOT (~) bitwise operation. Doing NOT (~) operation on a number toggles all of its bits.
#include <stdio.h>
void binary(unsigned int n)
{
unsigned int mask = 0x80000000;
while(mask) {
printf("%d", (n&mask)?1:0);
mask = mask >> 1;
}
}
void main()
{
unsigned int num = 0;
printf("Enter an integer: ");
scanf("%d", &num);
printf("Binary represntation of the input number:\n");
binary(num);
num = ~num;
printf("\n\nAfter toggling all bits of the number:\n");
binary(num);
printf("\n");
}
This program takes an unsigned integer as input and prints the 32-bits of that number. Then it does NOT (~) operation on the number to toggle all bits. After toggling it prints the bits of the number.
Here is the output.
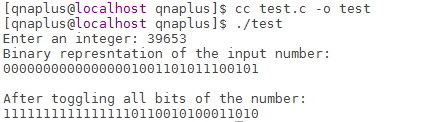
Toggle a Particular Bit
In the previous article I discussed about how to toggle a particular bit of a number in detail. Here we’ll see the program.
#include <stdio.h>
void binary(unsigned int n)
{
unsigned int mask = 0x80000000;
while(mask) {
printf("%d", (n&mask)?1:0);
mask = mask >> 1;
}
}
void main()
{
unsigned int num = 0;
int pos = 0;
printf("Enter an integer: ");
scanf("%d", &num);
printf("Binary represntation of the input number:\n");
binary(num);
printf("\nEnter a bit position to toggle (0-31): ");
scanf("%d", &pos);
num = num ^ (0x01 << pos);
printf("\n\nAfter toggling %d-th bit of the number:\n", pos);
binary(num);
printf("\n");
}
Here is the output.
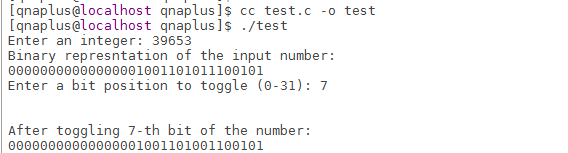
Toggle All but a Particular Bit
Say we want to toggle all bits of an number but the k-th bit should remain unchanged. To do that we have to first toggle the k-th bit and then toggle all bits. So the k-th would be toggled twice. That means that will remain unchanged. Other bits would be toggled once.
#include <stdio.h>
void binary(unsigned int n)
{
unsigned int mask = 0x80000000;
while(mask) {
printf("%d", (n&mask)?1:0);
mask = mask >> 1;
}
}
void main()
{
unsigned int num = 0;
int pos = 0;
printf("Enter an integer: ");
scanf("%d", &num);
printf("Binary represntation of the input number:\n");
binary(num);
printf("\nEnter a bit position that will remain unchanged (0-31): ");
scanf("%d", &pos);
num = ~(num ^ (0x01 << pos));
printf("\n\nAfter toggling all but %d-th bit of the number:\n", pos);
binary(num);
printf("\n");
}
Here is the output.
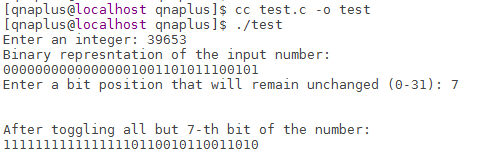
In this example, all bits of the input number toggled expect bit 7 (starting from 0)