In this article we saw how to check a particular bit of a number. Here we’ll see how to set, clear or toggle a bit of a number.
Setting a Bit
Setting a bit means making that bit 1 irrespective of the previous value of that bit.
We can use bitwise operation OR (|) to set a particular bit.
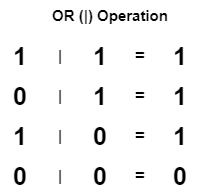
From the OR (|) operation chart, we can see that if we do OR (|) between 1 and any other bit, the result becomes 1. But if we do OR (|) operation between 0 and any other bit, the bit remains unchanged.
The binary representation of 39653 is 1001101011100101. Now say we want to set the 8-th (starting from 0) bit which is currently 0. First we have to find out a number whose 8-th bit is 1 and others are 0. If we do OR (|) with such a number, the 8-th will become 1 and others will remain unchanged.
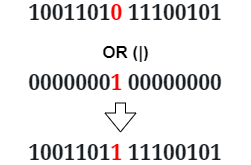
The Program
#include <stdio.h>
void binary(int n)
{
if(n > 0)
{
binary(n/2);
printf("%d", n%2);
}
}
void main()
{
int num = 0, pos = 0;
printf("Enter an integer: ");
scanf("%d", &num);
printf("Binary of %d: ", num);
binary(num);
printf("\nEnter a bit position to set: ");
scanf("%d", &pos);
num = num | (0x01 << pos);
printf("After setting %d-th bit, the number becomes:\n", pos);
printf("%d, Binary: ", num);
binary(num);
printf("\n");
}
This program takes an integer as input and prints that in binary form. Then it takes to bit position to set. It creates a number whose the specified bit is 1 and other bits are 0 (0x01 << pos). Then it did the OR (|) operation with the input number.
Here is the output.
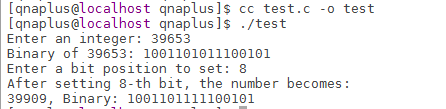
Clearing or Resetting a Bit
Clearing or setting a bit means making that bit 0 irrespective of the previous value of that bit.
We can use bitwise operation AND (&) to set a particular bit.
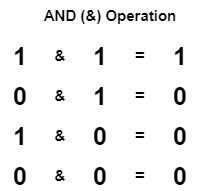
From the AND (&) operation chart, we can see that if we do AND (&) between 0 and any other bit, the result becomes 0. But if we do AND (&) operation between 1 and any other bit, the bit remains unchanged.
Here also we’ll use the same number 39653 (1001101011100101) as example. Here we’ll clear the 7-th (starting from 0) bit which is currently 1. First we have to find out a number whose 7-th bit is 0 and others are 1. If we do AND (&) with such a number, the 7-th will become 0 and others will remain unchanged.
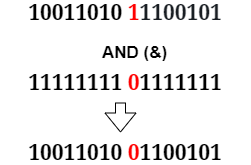
The Program
#include <stdio.h>
void binary(int n)
{
if(n > 0)
{
binary(n/2);
printf("%d", n%2);
}
}
void main()
{
int num = 0, pos = 0;
printf("Enter an integer: ");
scanf("%d", &num);
printf("Binary of %d: ", num);
binary(num);
printf("\nEnter a bit position to clear: ");
scanf("%d", &pos);
num = num & ~(0x01 << pos);
printf("After clearing %d-th bit, the number becomes:\n", pos);
printf("%d, Binary: ", num);
binary(num);
printf("\n");
}
This program takes an integer as input and prints that in binary form. Then it takes to bit position to clear. It creates a number whose the specified bit is 0 and other bits are 1 (~(0x01 << pos)). Then it did the AND (&) operation with the input number.
Here is the output

Toggling a Bit
Toggling a bit means altering the bit value. If the bit is 0, make it 1. If the bit is 1, make it 0.
We can use bitwise operation XOR (^) to toggle a particular bit.
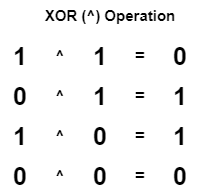
From the XOR (^) operation chart, we can see that if we do XOR (^) between 1 and any other bit, bit value alters. But if we do XOR (^) operation between 0 and any other bit, the bit remains unchanged.
So with our example number 39653 (1001101011100101), we’ll toggle the 7-th (starting from 0) bit which is currently 1. First we have to find out a number whose 7-th bit is 1 and others are 0. If we do XOR (^) with such a number, the 7-th will become 0 and others will remain unchanged.
If we do the same operation on 8-th bit, the bit will become 1 from 0.
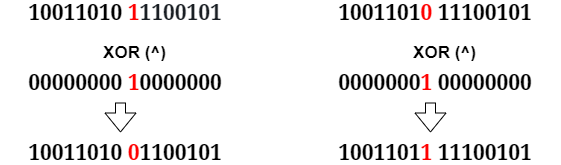
The Program
#include <stdio.h>
void binary(int n)
{
if(n > 0)
{
binary(n/2);
printf("%d", n%2);
}
}
void main()
{
int num = 0, pos = 0;
printf("Enter an integer: ");
scanf("%d", &num);
printf("Binary of %d: ", num);
binary(num);
printf("\nEnter a bit position to toggle: ");
scanf("%d", &pos);
num = num ^ (0x01 << pos);
printf("After toggling %d-th bit, the number becomes:\n", pos);
printf("%d, Binary: ", num);
binary(num);
printf("\n");
}
This program first prints the input number in binary form. Then it takes to bit position to set. It creates a number whose the specified bit is 1 and other bits are 0 (0x01 << pos). Then it did the XOR (^) operation with the input number.
Here is the output.
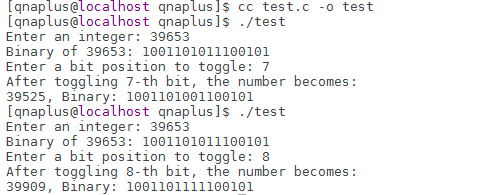