An integer consists of 32 bits. Here we’ll see how to write C program to check whether a particular bit is 0 or 1.
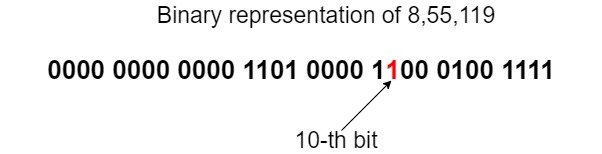
The above diagram shows the binary representation of an integer 8,55,119. Bit position will start from 0. That means the least significant bit position is 0 and the most significant bit position is 31. So the 10-th bit of the above integer is 1.
Program to Check a Bit
#include <stdio.h>
int check_bit(int val, int n) {
return ((val >> n) & 0x01);
}
int main(){
int x = 0;
int pos = 0;
printf("Enter an integer: ");
scanf("%d", &x);
printf("Enter position: ");
scanf("%d", &pos);
printf("%d-th position of %d is %d.\n", pos, x, check_bit(x, pos));
}
The check_bit() function returns the n-th bit of val. If n-th bit is 1, the function returns 1. Similarly if n-th bit is 0, the function returns 0.
The check_bit() function first brings the n-th bit to the 0-th position by shifting n bits (val >> n). If we do bitwise AND (&) operation with the shifted number, the result will be equal to the 0-th bit position.
Here is the output of the program.
Enter an integer: 855119
Enter position: 10
10-th position of 855119 is 1.
Enter an integer: 855119
Enter position: 9
9-th position of 855119 is 0.