In the previous article we saw how to remove an element of a particular position from an array. Here we’ll see how we can remove all occurrences of a number. For example, if an array has the number 100 three times, all three ocurrences of 100 will be removed. The holes created by the removal of three 100’s will be filled by other numbers in the array. As a result, the size of the array will be reduced by three.
Here is the C program.
#include <stdio.h>
int remove_val(int* arr, int size, int val) {
int i, count = 0;
for (i = 0; i < size; i++) {
if (arr[i + count] == val) count++;
arr[i] = arr[i+ count];
}
return size - count;
}
void print_array(int* arr, int size) {
int i;
for (i = 0; i < size; i++) {
printf(" %d", arr[i]);
}
printf("\n");
}
int main() {
int i, n, val, r;
int arr[128];
printf("Enter number of elements: ");
scanf("%d", &n);
/*Taking the array as input from user...*/
for (i = 0; i < n; i++) {
printf("Enter %d-th element: ", i);
scanf("%d", (arr+i));
}
/*Printing the input array...*/
printf("The input array: ");
print_array(arr, n);
printf("Enter a value to remove: ");
scanf("%d", &val);
r = remove_val(arr, n, val);
/*Printing the array after deletion...*/
printf("The array after removing %d: ", val);
print_array(arr, r);
return 0;
}
This program first takes an array as input and prints the array. Then it takes the number to be removed as input. It calls the remove_val() function to remove a particular number. This function returns the new size of the array. The it prints the array with the new size.
Here is the output of the program.
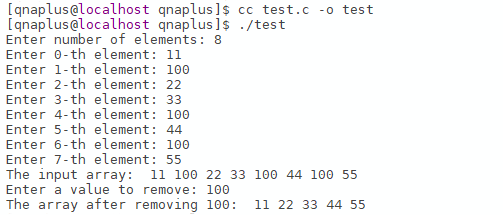