Here we’ll see how to delete an element of a particular position from an array. Deleting element means left-shifting the right elements starting from the position.
#include <stdio.h>
void delete_pos(int* arr, int size, int pos) {
int i;
/*If the position is greater than the array size, deletion not possible*/
if (pos >= size) {
printf("Deletion not possible.\n");
return;
}
for (i = pos; i < size - 1; i++) {
arr[i] = arr[i+1];
}
}
void print_array(int* arr, int size) {
int i;
for (i = 0; i < size; i++) {
printf(" %d", arr[i]);
}
printf("\n");
}
int main() {
int i, n, pos;
int arr[128];
printf("Enter number of elements: ");
scanf("%d", &n);
/*Taking the array as input from user...*/
for (i = 0; i < n; i++) {
printf("Enter %d-th element: ", i);
scanf("%d", (arr+i));
}
/*Printing the input array...*/
printf("The input array: ");
print_array(arr, n);
printf("Enter position: ");
scanf("%d", &pos);
delete_pos(arr, n, pos);
/*Printing the array after deletion...*/
printf("The array after deletion: ");
print_array(arr, n-1);
return 0;
}
The above program first takes the array as input and the position of an element to be deleted. Then it prints the array before and after deleting the element. The delete_pos() function is called to delete an element from a particular position.
Here is the output of the program.
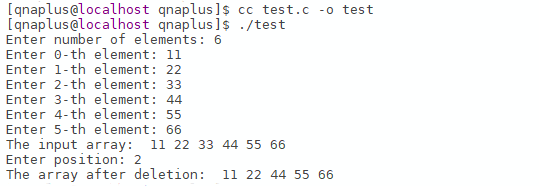
Read also: Insert element in an array.