Here we’ll see how to delete the last node of a linked list. The last node always points to NULL. To delete that, we have to traverse up to last node. We have to point the second last node to NULL and free memory of the last node. After deletion the second last node will become the new last node.
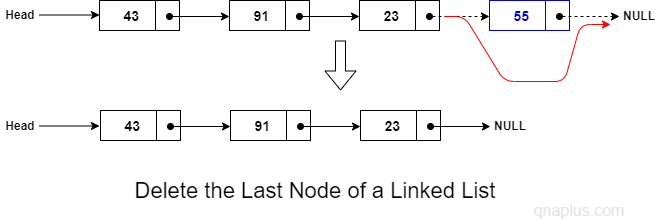
Logic to Delete the Last Node of a Linked List
- If head points to NULL, return. The linked list is empty – there is no node.
- If the first node points to NULL – there is only one node in the linked list, delete the first node and assign NULL to head.
- Point prev to first node and cur to second node.
- Keep moving prev and cur (prev = prev->next, cur = cur->next) until cur->next is NULL.
- If cur->next is NULL, set prev->next equals to NULL and delete cur.
Read also: Delete any node from a linked list.
Read also: Delete the first node of a linked list.
The Program
/*File: test.c*/
#include <stdio.h>
#include <stdlib.h>
struct node{
int val;
struct node *next;
};
/*Delete the last node of a linked list.*/
void delete_last_node(struct node **head) {
struct node *prev = NULL, *cur = NULL;
/*Linked list does not exist or the list is empty*/
if(head == NULL || *head == NULL) return;
/*If there is only one node in the list*/
if((*head)->next == NULL) {
free(*head);
*head = NULL;
}
prev = *head;
cur = prev->next;
while(cur->next) {
prev = prev->next;
cur = cur->next;
}
prev->next = NULL;
free(cur);
}
/*Print the linked list*/
void print_list(struct node *head) {
printf("H->");
while(head)
{
printf("%d->", head->val);
head = head->next;
}
printf("|||\n");
}
/*Insert an element at the front of the list*/
void insert_front(struct node **head, int value) {
struct node * new_node = NULL;
/*Allocating memory for the new node*/
new_node = (struct node *)malloc(sizeof(struct node));
if (new_node == NULL)
{
printf("Failed to insert element. Out of memory");
}
new_node->val = value;
/*Pointing the new node to where head is currently pointing to*/
new_node->next = *head;
/*Pointing head to new node.*/
*head = new_node;
}
void main()
{
int count = 0, i, val;
struct node * head = NULL;
printf("Enter number of elements: ");
scanf("%d", &count);
for (i = 0; i < count; i++)
{
printf("Enter %dth element: ", i);
scanf("%d", &val);
insert_front(&head, val);
}
printf("Initial Linked List: ");
print_list(head);
delete_last_node(&head);
printf("Linked List after last node deleted: ");
print_list(head);
}
This program first creates a linked list and then call the delete_last_node() function. The delete_last_node() takes a linked list a input and deletes the last node. Here we passed head as double pointer because head might get changed from inside the function if there is only one node in the list. To change a pointer from inside a function we need to pass that as double pointer.
Here is the output of the above program.
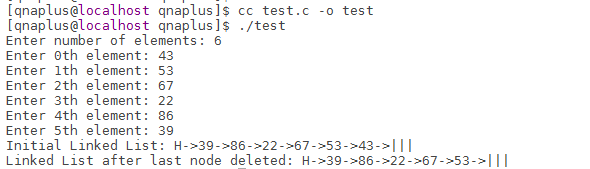