We often work with data structures with connected nodes – like trees and graphs. In a tree, the parent nodes generally hold the pointers of their child nodes. But the child nodes can also hold the pointer back to their parent. Developers often refer this type of pointers as backpointers.
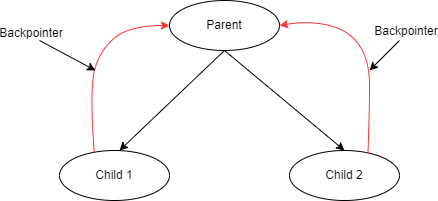
In this diagram, the black arrows are representing the normal forward pointers. In time of creating a child node, the parent node can pass its own pointer to the child node. Later, we can get hold of the parent node from the child using that pointer. The red lines are representing such type of back pointers.
Any object containing its creator’s pointer can be referred as containing a backpointer. The relationship does not have to be hierarchical.
Benefits of Backpointer
- Ease of traversing: Using backpointer we can traverse back and forth from parent to child and vice versa easily. For example, if all tree nodes hold their parents’ pointer, we can very easily traverse through the tree nodes easily.
- Accessing parent objects functionalities: The parent node does not have to be always the same type of node as the child. The parent node might have some functionalities (functions) the child might require for its own work. The child can get that via backpointers.
- Get access to parent’s properties: The child node can get access to the parent’s properties – value of member variables – though the backpointer.
Backpointer Implementation
#include <iostream>
using namespace std;
class node {
public:
node(int val, node *parent = NULL) {
val_ = val;
parent_ = parent;
left_child_ = right_child_ = NULL;
}
~node() {
if (left_child_) delete left_child_;
if (right_child_) delete right_child_;
}
node *addLeftChild(int val) {
left_child_ = new node(val, this);
return left_child_;
}
node *addRightChild(int val) {
right_child_ = new node(val, this);
return right_child_;
}
int getVal() {
return val_;
}
int getParentVal() {
if (parent_ == NULL) return 0;
return parent_->getVal();
}
node *getParent() {
return parent_;
}
private:
int val_;
node *parent_;
node *left_child_;
node *right_child_;
};
int main() {
node *root = new node(10);
node *child1 = root->addLeftChild(20);
node *child2 = root->addRightChild(30);
node *parent = child1->getParent();
cout << "Parent value: " << parent->getVal() << endl;
cout << "Parent value from child1: " << child1->getParentVal() << endl;
return 0;
}
$ g++ -o test test.cpp
$ ./test
Parent value: 10
Parent value from child1: 10
In this example, we have a class, node, that can be used to construct a binary tree. It has two pointers for its two child nodes and another pointer, parent_, to hold the parents address.
The addLeftChild() or the addRightChild() function creates a child node and returns that child’s pointer. In time of creatine the child, it passes its own pointer, referred as this, to this child. The ways the child possesses it parent’s or creator’s pointer.
Now, if we have access to a child, we can easily traverse to its parent. The getParent() function returns the address of the parent. We can get the value of the parent from the returned address. The first ‘cout‘ statement showed that.
The child can also directly access the value of the parent though the backpointer, parent_. The getParentVal() function returns the parent’s value. Inside that function, the child node accessed its parent’s value via the backpointer, parent_. That’s why the second ‘cout’ state printed the same value.