Here we’ll see how to insert an element in an array at a particular position, after or before an existing element. If we insert an element in an array, all the elements, after the inserted position, need to be shifted to the next position.
Inserting at a Particular Position
We’ll start by inserting a new element at a particular position of the array.
#include <stdio.h>
void insert_at(int val, int pos, int* arr, int size) {
int i;
/*If the insert position is greater than the array size, insert at the end of the array*/
if (pos >= size) {
arr[size] = val;
return;
}
for (i = size; i > 0; i--) {
arr[i] = arr[i-1];
if (pos == i-1) {
arr[i-1] = val;
break;
}
}
}
void print_array(int* arr, int size) {
int i;
for (i = 0; i < size; i++) {
printf(" %d", arr[i]);
}
printf("\n");
}
int main() {
int i, n, val, pos;
int arr[128];
printf("Enter number of elements: ");
scanf("%d", &n);
/*Taking the array as input from user...*/
for (i = 0; i < n; i++) {
printf("Enter %d-th element: ", i);
scanf("%d", (arr+i));
}
/*Printing the input array...*/
printf("The input array: ");
print_array(arr, n);
printf("Enter a value to be inserted: ");
scanf("%d", &val);
printf("Enter position: ");
scanf("%d", &pos);
insert_at(val, pos, arr, n);
/*Printing the array after insertion...*/
printf("The array after insertion: ");
print_array(arr, n+1);
return 0;
}
This program first takes an array of integers as input and prints that. Then it takes input of the new element to be inserted and the position. It then calls the insert_at() function to insert the new element into the existing array.
The insert_at() function takes the new element and its postion and the existing array as input. If the position (pos) is greater than the array size, then it inserts the new element at the end of the array and returns. If not a loop starts from the end of the array. The iterrator, i, starts from size, where last element position is (size -1). In every iteration, (i-1)-th element is copied to i-th position. If the position of the new elemet is (i-1), then the new element is copied to that position and the loop terminates.
After calling the insert_at() function, the program prints the new array. After the insertion, the array size increases by one. That’s why, it prints (n+1) elements.
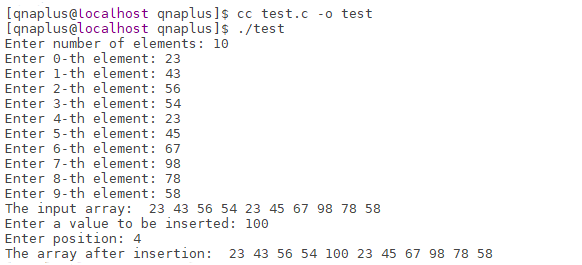
From this output, we can see that a new element (100) is inserted at 4-th (starting from 0) position.
Inserting After an Existing Element
Here we’ll see how to insert a new element after an existing one. For example, if we want to insert the new element, 100, after the existing element 22, then 1oo will be insterted after all occurences of 22. That means array size can increase by more than one.
#include <stdio.h>
void insert_at(int val, int pos, int* arr, int size) {
int i;
/*If the insert position is greater than the array size, insert at the end of the array*/
if (pos >= size) {
arr[size] = val;
return;
}
for (i = size; i > 0; i--) {
arr[i] = arr[i-1];
if (pos == i-1) {
arr[i-1] = val;
break;
}
}
}
int insert_after(int val, int after, int* arr, int size) {
int i;
int size1 = size;
for (i = 0; i < size1; i++) {
if (arr[i] == after) {
insert_at(val, i+1, arr, size1);
size1++;
}
}
return size1;
}
void print_array(int* arr, int size) {
int i;
for (i = 0; i < size; i++) {
printf(" %d", arr[i]);
}
printf("\n");
}
int main() {
int i, n, val, after, size;
int arr[128];
printf("Enter number of elements: ");
scanf("%d", &n);
/*Taking the array as input from user...*/
for (i = 0; i < n; i++) {
printf("Enter %d-th element: ", i);
scanf("%d", (arr+i));
}
/*Printing the input array...*/
printf("The input array: ");
print_array(arr, n);
printf("Enter a value to be inserted: ");
scanf("%d", &val);
printf("Insert after: ");
scanf("%d", &after);
size = insert_after(val, after, arr, n);
/*Printing the array after insertion...*/
printf("The array after insertion: ");
print_array(arr, size);
return 0;
}
Here we’ll use the insert_at() function. The new insert_after() function first finds the position where the new element to be inserted at. Then it calls the insert_at() function. The loop in insert_after() function checks whether the current element (arr[i]), matches the value after which the new element will be inserted. If it matches, then the insert_at() function is called with (i+1). The new element will be inserted at (i+1)-th position.
For every insert_at() call, then array size (size1) increases by one. After looping through all the elements, the insert_after() function returns the new array size. The main() function uses the new array size to print all elements in the array.
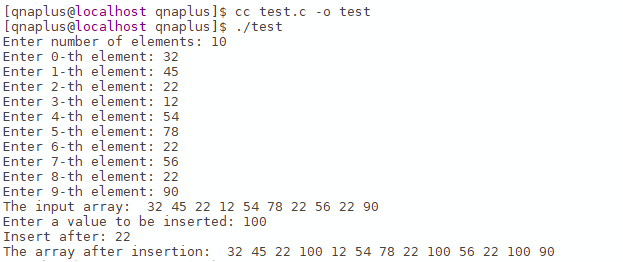
In this output, the new element 100 is inserted after 22. As there were 3 occurences of 22, the new element was inserted thrice in the array after 22.
Inserting After an Exiting Element
Inserting the new element before an existing one is very similar to the previous example. The insert_before() function is shown here.
int insert_before(int val, int before, int* arr, int size) {
int i;
int size1 = size;
for (i = 0; i < size1; i++) {
if (arr[i] == before) {
insert_at(val, i, arr, size1);
size1++;
i++;
}
}
return size1;
}
This function is similar to the insert_after() function except it call the insert_at() function with i instead of (i+1). One more differnce – the iterator, i, is also increased after insert_at() call. Because after insertion, the i-th element will move the (i+1)-th position. So we need to increment the iterator by 2 in this case. Otherwise, the checking (arr[i] == before) will continue to be true and the loop will become infinte. It will eventually lead to program crash.
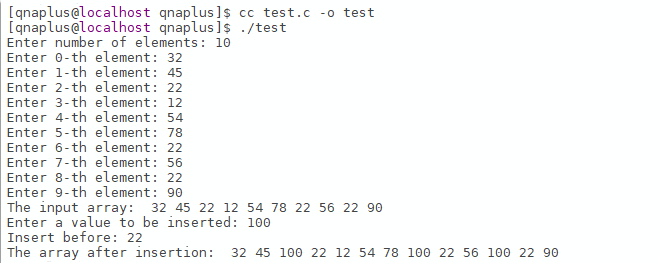
In this output we can see the new element 100 is inserted before all three accurences of 22.
Note: In all above examples, it is assumed that the array has enough allocated space (memory) to fit the new elements.