In the previous article, we saw how to check whether two strings are equal. But there the comparision was case sensitive. That means, ‘QnAPlus’ and ‘qnaplus’ are not equal. Here we’ll see how we can compare strings in a case insensitive way.
There is no library function in standard C to compare strings in case insensitive way. So we have to write our own function. Here is the program.
#include <stdio.h>
/*
Returns 1 if two input strings are equal (case insensitive), otherwise, 0.
*/
int is_string_equal(char* s1, char* s2) {
int i = 0;
while (s1[i] != '\0' && s2[i] != '\0') {
if (toupper(s1[i]) != toupper(s2[i])) return 0; /*i-th character is different*/
i++;
}
if (s1[i] == '\0' && s2[i] == '\0') return 1;
return 0;
}
int main() {
char s1[100], s2[100];
printf("Enter string1: ");
gets(s1);
printf("Enter string2: ");
gets(s2);
if (is_string_equal(s1, s2)) {
printf("Two strings are equal.\n");
} else {
printf("Two strings are not equal.\n");
}
return 0;
}
This program takes two strings as input and calls the is_string_equal() function to check whether they are equal. The the two input strings are eqaul, then the is_string_equal() function returns 1, otherwise, 0.
Here is the output of the program.
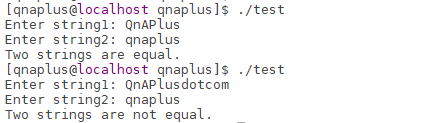