Here we’ll see how to check whether two strings are equal. C string (string.h) library already provides a function to do that.
Using strcmp()
- Take two strings as input (say, s1 and s2).
- Call strcmp() with two input strings.
- If strcmp() returns 0, the strings are equal, otherwise, not.
#include <stdio.h>
#include <string.h>
int main() {
char s1[100], s2[100];
printf("Enter string1: ");
gets(s1);
printf("Enter string2: ");
gets(s2);
if (strcmp(s1, s2) == 0) {
printf("Two strings are equal.\n");
} else {
printf("Two strings are not equal.\n");
}
return 0;
}
Here is the output of the program.
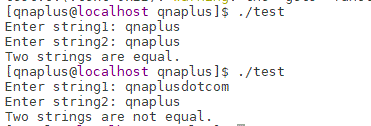
Not Using strcmp()
In the above example, we used the library function, strcmp(), to compare the strings. But we can write our own function for the same purpose.
Logic to Check Whether Two Strings are Equal
- Traverse two strings from the 0-th position until the character of at least one string is NULL (‘\0’).
- If the characters of a particular position of two strings are not equal, return 0. Strings are not equal.
- After traversal, if the characters of the current position of both strings are NULL (‘\0’), return 1. Strings are equal. Otherwise, return 0.
Here is the program.
#include <stdio.h>
/*
Returns 1 if two input strings are equal, otherwise, 0.
*/
int is_string_equal(char* s1, char* s2) {
int i = 0;
while (s1[i] != '\0' && s2[i] != '\0') {
if (s1[i] != s2[i]) return 0; /*i-th character is different*/
i++;
}
if (s1[i] == '\0' && s2[i] == '\0') return 1;
return 0;
}
int main() {
char s1[100], s2[100];
printf("Enter string1: ");
gets(s1);
printf("Enter string2: ");
gets(s2);
if (is_string_equal(s1, s2)) {
printf("Two strings are equal.\n");
} else {
printf("Two strings are not equal.\n");
}
return 0;
}
Here the is_string_equal() function returns 1 if two inpur strings are equal, otherwise, returns 0.
In the above programs, the string comparision is case sensitive. That means, ‘QnAPlus’ and ‘qnaplus’ are not equal. Here is how we can compare strings in case insensitive way.