Here we’ll see how to write C program to left or right rotate bits of a number. For example, an unsigned integer consists of 32-bit. Number 2591458749 is represented as 10011010011101101000010110111101 in binary. The diagram below shows how the bits of that number will look like after rotating 8 bits.
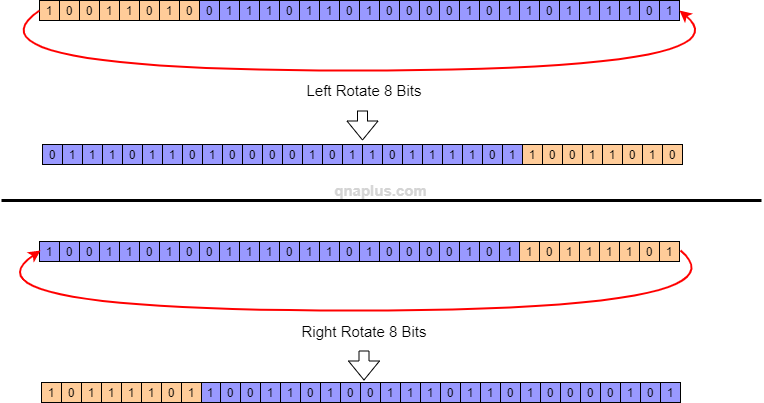
Logic to Left Rotate Bits
Say we want to left rotate an integer of size 32 bits by n bits.
- Create a number, num1 by left shifting n bits. (num1 = num << n)
- Create another number, num2, by right shifting (32-n) bits. (num2 = num >> (32-n))
- The result is num1 OR (|) num2.
Logic to Rotate Rotate Bits
Logic is very similar if we want to right rotate an integer of size 32 bits by n bits.
- Create a number, num1 by right shifting n bits. (num1 = num >> n)
- Create another number, num2, by left shifting (32-n) bits. (num2 = num << (32-n))
- The result is num1 OR (|) num2.
The Program
#include <stdio.h>
void binary(unsigned int n)
{
unsigned int mask = 0x80000000;
while(mask) {
printf("%d", (n&mask)?1:0);
mask = mask >> 1;
}
}
unsigned int left_rotate(unsigned int num, int n) {
return (num << n) | (num >> (32 - n));
}
unsigned int right_rotate(unsigned int num, int n) {
return (num >> n) | (num << (32 - n));
}
void main()
{
unsigned int num = 0, result;
int n = 0;
printf("Enter an integer: ");
scanf("%d", &num);
printf("Binary represntation of the input number:\n");
binary(num);
printf("\nHow many bits to rotate (0-31)?: ");
scanf("%d", &n);
result = left_rotate(num, n);
printf("\n\nAfter left rotating %d bits:\n", n);
binary(result);
result = right_rotate(num, n);
printf("\n\nAfter right rotating %d bits:\n", n);
binary(result);
printf("\n");
}
This program takes an integer as input and prints the binary form of that number. Then it takes the input of how many bits to rotate. It rotates the number using left_rotate() and right_rotate() functions.
Here is the output.
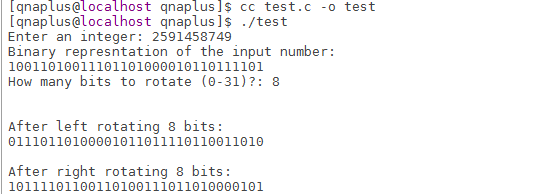