In Object Oriented Programming, inheritance is a mechanism of acquiring properties and behaviors in one class from another. In C++, one class can be derived from another class which is called base class. The derived class is often referred as a ‘child‘ class and the base one as ‘parent‘ class. It is like, the ‘child‘ inherits the properties and behaviors from the ‘parent‘.
This served the purpose of code re-usability in object oriented programming.
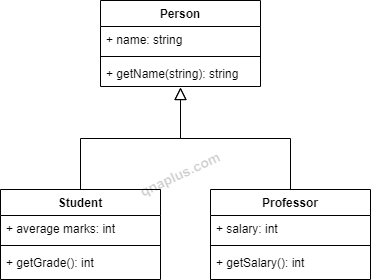
In this example, the parent class, Person, has one property, name and one behavior or function, getName().
Two classes, Student and Professor, are derived from the base class, Person. The Student class has its own property like, average marks and function, getGrade(). Similarly, the Professor class also has its own property, salary, and function getSalary().
At the same time both the derived classes will have the properties and functions of the base class. The Student class will have property, name, and function, getName(). Same thing is true for the Professor class.
In this next section, we’ll see inheritance in C++ code.
C++ Inheritance Code
#include <iostream>
using namespace std;
class Person {
public:
Person(string name) {
name_ = name;
}
string getName() {
return name_;
}
protected:
string name_;
};
class Student : public Person {
public:
Student(string name, int marks) : Person (name) {
avg_marks_ = marks;
}
int getGrade () {
if (avg_marks_ > 50) return 1;
return 0;
}
void printName() {
cout << name_ << endl;
}
private:
int avg_marks_;
};
class Professor : public Person {
public:
Professor(string name) : Person(name) {
salary_ = 100;
}
int getSalary() {
return salary_;
}
private:
int salary_;
};
int main() {
Person p1("Jack");
cout << "Name of the Person: " << p1.getName() << endl;
cout << endl;
Student s1("Harry", 82);
cout << "Name of the Student: " << s1.getName() << endl;
cout << "Grade of the Student: " << s1.getGrade() << endl;
s1.printName();
return 0;
}
$ g++ -o test test.cpp
srikanta@ubuntu:~/galaxy/ss/del$ ./test
Name of the Person: Jack
Name of the Student: Harry
Grade of the Student: 1
Harry
The example class structure is presented in C++ code. Here, Person is the base class that has the property, name_ and the function getName(). The Student class is derived from this base class.
Notice that the printName() function of Student class accessed the property, name_, which is inherited from the Person class.
In the main() function, we first created an object, p1, of type Person. We called the function getName() to get the name of the person, p1.
Then we created another object, s1, of type Student. We called the same getName() function to get the student name. But the getName() function is not its own function. It is inherited from the base class, person. The we called the printName() function to demonstrate that a member function of derived class can access a member variable of the base class.
Not everything from the base class can be inherited to the derived class.
- The constructor and the destructor of the base class are not inherited to the derived class – even though though they can be called from the derived class.
- The overloaded operators of the base class are not inherited to the derived class.
- The private members of the base class are also not inherited the derived class.
Another important thing to notice here is that the Person class is derived in public mode.
There are 3 modes of inheritance:
- Public: Public members of the base class becomes public and the protected members of the base class become protected in derived class.
- Protected: Both the public and the protected members of the base class become protected in the derived class.
- Private: Both the public and the protected members of the base class become private in the derived class.