An application takes inputs in various ways. It can ask for input from user while it is running. It can read something from file or socket. Another way is, we can give inputs in time of starting the application in the form of command line arguments. For example, when we run Linux commands we give several options as command line arguments to application . Here we’ll see how to write a C program that will take command line arguments.
#include <stdio.h>
int main( int argc, char *argv[] ) {
int i = 0;
printf("Application name: %s\n\n", argv[0]);
printf("Command line arguments:\n");
for(i = 1; i < argc; i++) {
printf("Argument %d: %s\n", i, argv[i]);
}
return 0;
}
Here the main() function will have two parameters, argc and argv. When the program starts, argc contains the number of arguments passed, including the application name. The other parameter, argv, is an array of string. It holds all the command line arguments. The first element is always the application name.
Here is the output of the above program.
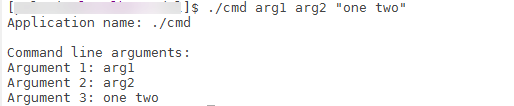