Here we’ll see how to write C program to find the minimum and maximum numbers in an array.
#include <stdio.h>
int main()
{
int arr[128];
int n = 0, i = 0, min = 0, max = 0;
printf("Enter the number of elements of the array: ");
scanf("%d", &n);
for(i = 0; i < n; i++) {
printf("arr[%d] = ", i);
scanf("%d", &arr[i]);
}
min = arr[0];
max = arr[0];
for(i = 1; i < n; i++) {
if(arr[i] < min) {
min = arr[i];
} else if (arr[i] > max) {
max = arr[i];
}
}
printf("Minimum number in the array: %d.\n", min);
printf("Maximum number in the array: %d.\n", max);
return 0;
}
Here we assigned the first element of the array to both minimum (min) and maximum (max). Then we traverse the array from the second element to the last element. If any element is smaller than the current min value, then that element is assigned to min. Similarly, if an element is greater than the current max, then that element is assigned to max.
Here is the output of the program.
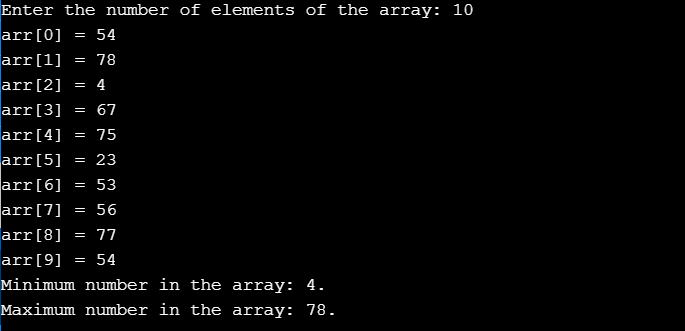