We can not copy an array using simple assignment operation like any other primitive data types. Here we’ll discuss the techniques to copy an array from one to another.
By Traversing
We can copy an array by traversing the source array – coping one element in each iteration. This is simplest, most common and widely used mechanism.
/*test.c*/
#include <stdio.h>
void print_array(int* arr, int size) {
int i = 0;
for (i = 0; i < size; i++) {
printf("%d ", arr[i]);
}
printf("\n");
}
int main(){
const int MAX_SIZE = 32;
int size = 0;
int arr_src[MAX_SIZE];
int arr_dst[MAX_SIZE];
int i = 0;
printf("Enter size: ");
scanf("%d", &size);
printf("Enter the array elements:\n");
for(i = 0; i < size; i++) {
printf("arr[%d] = ", i);
scanf("%d", arr_src + i);
}
printf("\nThe entered array:\n");
print_array(arr_src, size);
/* Copying the array */
for(i = 0; i < size; i++) {
arr_dst[i] = arr_src[i];
}
printf("The copied array:\n");
print_array(arr_dst, size);
}
Here we first take input of the source array. Then we traversed the array and in every iteration we copied one element from source array to the destination. Array elements are referred by index i. We printed the input array before copying. After copying that to the destination array, we printed the destination array.
Here is the output.
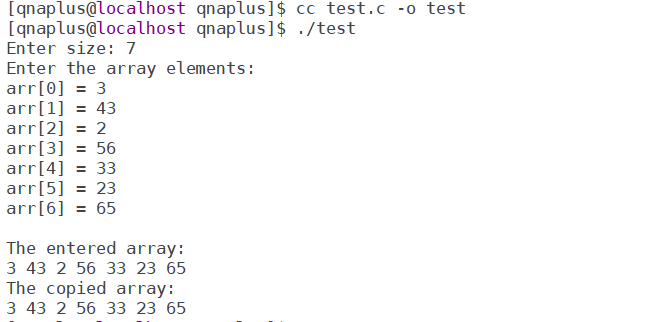
Using Memory Copy
Array elements are stored in consecutive memory locations. Instead of traversing the array, we can copy the whole array in one memory copy operation.
/*test.c*/
#include <stdio.h>
#include <memory.h>
void print_array(int* arr, int size) {
int i = 0;
for (i = 0; i < size; i++) {
printf("%d ", arr[i]);
}
printf("\n");
}
int main(){
const int MAX_SIZE = 32;
int size = 0;
int arr_src[MAX_SIZE];
int arr_dst[MAX_SIZE];
int total_size = 0;
int i = 0;
printf("Enter size: ");
scanf("%d", &size);
printf("Enter the array elements:\n");
for(i = 0; i < size; i++) {
printf("arr[%d] = ", i);
scanf("%d", arr_src + i);
}
printf("\nThe entered array:\n");
print_array(arr_src, size);
/* Copying the array */
total_size = size * sizeof(int);
memcpy(arr_dst, arr_src, total_size);
printf("The copied array:\n");
print_array(arr_dst, size);
}
Here first we calculated the total size (total_size) of the array. The array has size elements. Each element is an integer. So the total size is size * sizeof(int).
In the next statement we copied the whole array of size total_size using memcpy.
Output of this program would be exactly same as the first program.