Here we’ll see how to write a C program to compare two linked lists. First we’ll create three linked lists, first two are equal and third one is different. We’ll compare these linked lists using the check_equal() function.
#include <stdio.h>
#include <stdlib.h>
struct node{
int val;
struct node *next;
};
int check_equal(struct node *head1, struct node *head2) {
while(head1 != NULL && head2 != NULL) {
if(head1->val != head2->val) return 0;
head1 = head1->next;
head2 = head2->next;
}
if (head1 != NULL) return 0;
if (head2 != NULL) return 0;
return 1;
}
void print_list(struct node *head)
{
printf("H->");
while(head)
{
printf("%d->", head->val);
head = head->next;
}
printf("|||\n\n");
}
void insert_front(struct node **head, int value)
{
struct node * new_node = NULL;
new_node = (struct node *)malloc(sizeof(struct node));
if (new_node == NULL)
{
printf("Failed to insert element. Out of memory");
}
new_node->val = value;
new_node->next = *head;
*head = new_node;
}
int main()
{
struct node * head1 = NULL;
struct node * head2 = NULL;
struct node * head3 = NULL;
/*Creating the first linked list*/
insert_front(&head1, 16);
insert_front(&head1, 83);
insert_front(&head1, 89);
insert_front(&head1, 12);
insert_front(&head1, 67);
insert_front(&head1, 20);
/*Creating the second linked list*/
insert_front(&head2, 16);
insert_front(&head2, 83);
insert_front(&head2, 89);
insert_front(&head2, 12);
insert_front(&head2, 67);
insert_front(&head2, 20);
/*Creating the third linked list*/
insert_front(&head3, 16);
insert_front(&head3, 83);
insert_front(&head3, 50);
insert_front(&head3, 12);
insert_front(&head3, 67);
insert_front(&head3, 20);
printf("Linked List 1: ");
print_list(head1);
printf("Linked List 2: ");
print_list(head2);
printf("Linked List 3: ");
print_list(head3);
printf("\nChecking Linked List 1 and Linked List 2...\n");
if(check_equal(head1, head2)) {
printf("Linked List 1 and Linked List 2 are equal.\n");
} else {
printf("Linked List 1 and Linked List 2 are not equal.\n");
}
printf("\nChecking Linked List 1 and Linked List 3...\n");
if(check_equal(head1, head3)) {
printf("Linked List 1 and Linked List 3 are equal.\n");
} else {
printf("Linked List 1 and Linked List 3 are not equal.\n");
}
return 0;
}
The check_equal() function traverses the linked lists until at least one of them reaches to NULL (end). If the value fields of two linked list are not equal at any point, the function returns 0 (not equal).
After traversal, if any one list does not reach to NULL (end), then it also returns 0. Next two lines check that. If both lists reach to NULL after traversal and values of all nodes are equal, it returns 1 (equal).
Here is the output of the program.
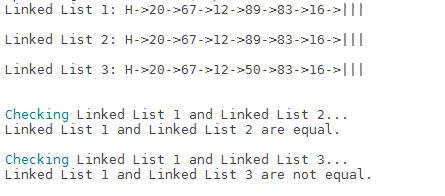
Recursive Version
Here is the recursive function of the check_equal() function.
int check_equal(struct node *head1, struct node *head2) {
if (head1 == NULL && head2 == NULL) return 1;
if(head1 != NULL && head2 != NULL) {
if(head1->val != head2->val) return 0;
return check_equal(head1->next, head2->next);
}
return 0;
}